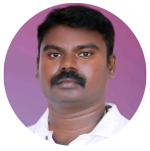
Cloud Technologist | Architecting Innovative Solutions for Digital Transformation | AWS, Azure, GCP Expert | Passionate about Emerging Technologies
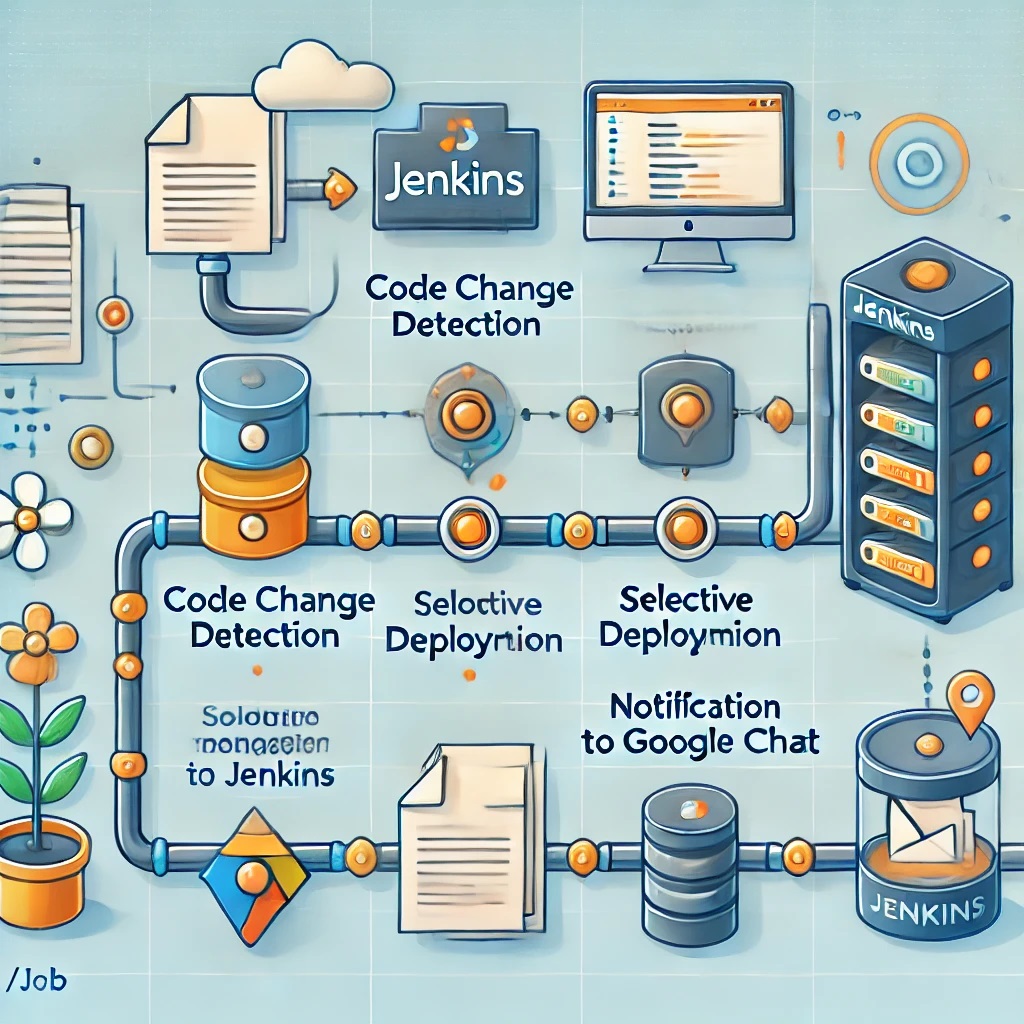
Introduction
Jenkins, combined with Groovy scripting, provides a powerful way to automate selective deployments based on specific folder changes in your repository. This blog will walk you through the creation of a Jenkins pipeline script using Groovy, designed to deploy only the necessary components, send notifications to Google Chat, and manage the build process efficiently.
Why Selective Deployment Matters
Selective deployment allows you to focus resources on only those components that have been modified, reducing build times and minimizing the risk of introducing errors in unrelated parts of the application. This is particularly important in environments where multiple applications or services are managed within the same repository.
Step-by-Step Pipeline Implementation
Let’s dive into the Groovy script and understand how each part works to achieve targeted deployment.
1. Setting Up the Environment
The environment block sets up the necessary paths and variables for the pipeline. This includes setting up the JAVA_HOME, adding it to the PATH, and defining variables for Google Chat notifications and Git commands.
environment {
JAVA_HOME = "/usr/lib/jvm/java-17-openjdk-amd64"
PATH = "${env.JAVA_HOME}/bin:${env.PATH}"
GCHAT_WEBHOOK_URL = 'https://chat.googleapis.com/v1/spaces/xxxxxxxxxx/messages?key=xxxxxxxxxxxxxx'
GIT_LAST_AUTHOR = sh(script: 'git --no-pager show -s --format=\'%an\' $GIT_COMMIT', returnStdout: true).trim()
GIT_LAST_COMMIT = sh(script: 'git log -1 --pretty=\'%B\'', returnStdout: true).trim()
LOCAL_PATH ="${WORKSPACE}"
BUILD_NUMBER="${BUILD_NUMBER}"
}
2. Initial Setup and Source Code Management (SCM)
The pipeline starts with the Java version setup and checks out the latest code from the repository.
stage('Setup Java') {
steps {
sh "java -version"
}
}
stage('SCM') {
steps {
checkout scm
}
}
3. Managing Build Stop and Start Notifications
We ensure that only the most recent build is active, using Jenkins milestones to avoid overlapping builds. Additionally, a notification is sent to Google Chat at the start of the build, containing the build status, commit details, and author.
stage('Stop Old Build') {
steps {
script {
milestone label: 'Stop Old Builds', ordinal: Integer.parseInt(env.BUILD_ID) - 1
milestone label: 'Current Build', ordinal: Integer.parseInt(env.BUILD_ID)
}
}
}
stage('Start Notification') {
steps {
script {
googlechatnotification message: "Build started: ${currentBuild.currentResult}\\n Job: *${env.JOB_NAME}*\\n BuildNo: ${env.BUILD_NUMBER}\\n Last commit: *${env.GIT_LAST_COMMIT}*\\n Author: *${env.GIT_LAST_AUTHOR}*", notifySuccess: true, url: env.GCHAT_WEBHOOK_URL
}
}
}
4. Building the Maven Project
The Maven build is triggered only if the current build status is successful or hasn’t encountered any issues yet.
stage('Maven Build') {
when {
expression {
currentBuild.result == null || currentBuild.result == 'SUCCESS'
}
}
steps {
sh "mvn clean install -Pbeta -DskipTests=true"
}
}
5. Parallel Deployment Based on Folder Changes
The deployment process is handled in parallel stages, where each stage checks if there are changes in the respective folders (folder1, folder2, and the root directory). If changes are detected, the corresponding application is deployed.
stage('Parallel Directory specific deployment') {
parallel {
stage('folder1 Deploy') {
steps {
script {
def changes = sh(script: 'git diff --name-only HEAD^ HEAD', returnStdout: true).trim()
if (changes.contains('jobs/folder1/')) {
sh "echo 'folder1 Deployment starting'"
} else {
echo "No folder1 Deployment"
}
}
}
}
stage('folder2 Deploy') {
steps {
script {
def changes = sh(script: 'git diff --name-only HEAD^ HEAD', returnStdout: true).trim()
if (changes.contains('jobs/folder2/')) {
sh "echo 'folder2 Deployment starting'"
} else {
echo "No folder2 Deployment"
}
}
}
}
stage('Root folder Deploy') {
steps {
script {
def changes = sh(script: 'git diff --name-only HEAD^ HEAD', returnStdout: true).trim()
if (changes.any { !it.contains('jobs/folder1/') && !it.contains('jobs/folder2/') }) {
sh "echo 'Root Deployment starting'"
} else {
echo "No Root Deployment"
}
}
}
}
}
}
6. Final Notifications
Upon completion of the build, a final notification is sent to Google Chat, providing detailed information about the build status, commit, and author. This ensures that the team is always informed of the build outcomes.
post {
always {
script {
googlechatnotification message: "Build ${currentBuild.currentResult}:\\n Job: *${env.JOB_NAME}*\\n BuildNo: ${env.BUILD_NUMBER}\\n Last commit: *${env.GIT_LAST_COMMIT}*\\n Author: *${env.GIT_LAST_AUTHOR}*\\n Full details: ${env.BUILD_URL}", url: env.GCHAT_WEBHOOK_URL
}
}
}
Conclusion
This Groovy-based Jenkins pipeline efficiently manages selective deployments, ensuring that only the necessary components are built and deployed based on the changes detected in specific folders. By automating notifications and handling build processes through targeted stages, this approach enhances both productivity and transparency in your CI/CD pipeline.
Further Enhancements
Consider adding the following to further improve your pipeline:
- Enhanced Error Handling: To automatically recover or notify in case of failures.
- Customizable Notifications: Integrate with more communication platforms or customize the message formats.
- Extended Deployment Options: For more complex deployment scenarios involving multiple environments or additional validation steps.
This method of automating and optimizing your deployment process with Groovy scripting in Jenkins can greatly benefit teams managing complex projects, ensuring smooth and efficient operations.